Google Drive Links: Workspace Add-on
My first approach to getting multiple Google Drive links.
Previously: Problem: Google Drive Links
My first approach was to create a Google Workspace Addon for Google Drive so that I could select some documents and get the links.
Google's documentation is not super-helpful, so I tried asking ChatGPT which kept suggesting that I use Google Sheets to make the add on (also not helpful).
It turns out you can make a sidebar for Google Drive, but it has some very particular limitations.
Demo #
- Code
- Install (coming soon, hopefully)
CardService
API #
First, you must use the Card
API to build up your UI. I'm not surprised that Google has an entirely different way to build up widgets from any of the other ways there are to build up widgets, but I kept looking for a way to just use HTML. After reading this helpful tutorial, I finally figured out how to get started and later discovered Google's Card-based interfaces for a better intro. Much later, I also found this add-ons intro that really helps clarify how to make an add-on that works in multiple contexts (I may revisit this later).
Second, you need to understand the difference between "non-contextual" (before you click on stuff) and "contextual" (after you click) modes. The entire UI is rebuilt every time something changes and it depends on whether the user has clicked on stuff or not. Because every click redraws the entire UI be careful about expensive function calls in the UI-drawing.
Third, you may use basic HTML in some of the text widgets. But "basic" here is more basic that I imagined:
- No
<ul>
elements. - No
style
attributes. - No wrapping of
<a href>
in<font color>
.
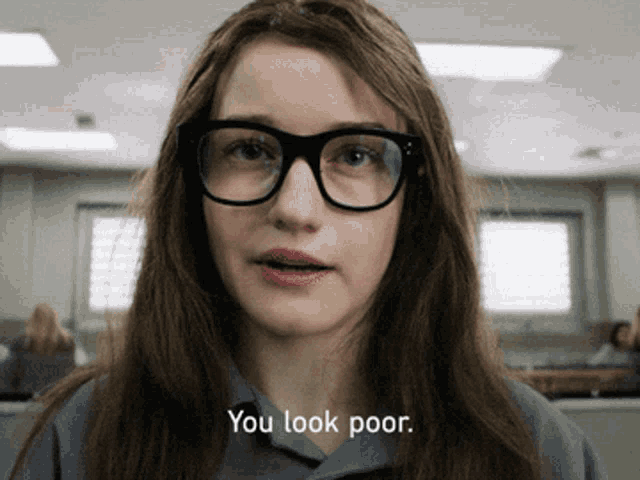
Finally, because the whole thing takes place in a pretty standard Google Apps Script environment, there's no access to the clipboard. I had hoped the new ctrl+c, ctrl+v
in Google Drive would mean that there's some way to copy stuff to the clipboard, but nope. Luckily for me, you can copy the generated HTML from the sidebar and paste (the non-underlined-and-weirdly-bright, but still blue) items into Gmail.
Home Screen #
The first step is to create a Google App Script.
The home screen for this sidebar will simply have some text saying to click on things:
const onHomepage = () => {
const card = CardService.newCardBuilder();
const section = CardService.newCardSection();
const text = CardService.newTextParagraph().setText(
"Select some files to view links."
);
section.addWidget(text);
card.addSection(section);
return card.build();
};
Pretty verbose, but meh.
Selected Items Screen #
When the user selects one or more items in Google Drive, we're going to show them a list of links. Unfortunately, we're limited to using <a>
and <br>
tags.
const getLink = (item) =>
`<a href="${DriveApp.getFileById(item.id).getUrl()}">${item.title}</a>`;
const onDriveItemsSelected = (e) => {
const card = CardService.newCardBuilder();
const section = CardService.newCardSection();
const links = e.drive.selectedItems.map(getLink);
section.addWidget(CardService.newTextParagraph().setText(links.join("<br>")));
card.addSection(section);
return card.build();
};
Exposing the Screens #
To make these screens appear at the appropriate times, we need to update the script metadata.
Click on the Project Settings
gear on the left-hand side and ensure Show "appsscript.json" manifest file in editor
is enabled. We'll come back here before publishing to enable the Google Cloud Project platform.
Switch back to the Editor
and look at appsscript.json
:
{
"timeZone": "America/New_York",
"dependencies": {},
"exceptionLogging": "STACKDRIVER",
"runtimeVersion": "V8"
}
Let's add the OAuth scope for reading metadata and the additional bits we need for the add-on:
{
"timeZone": "America/New_York",
"dependencies": {},
"exceptionLogging": "STACKDRIVER",
"runtimeVersion": "V8",
"oauthScopes": [
"https://www.googleapis.com/auth/drive.addons.metadata.readonly",
"https://www.googleapis.com/auth/drive.readonly"
],
"addOns": {
"common": {
"name": "Copy Links",
"logoUrl": "https://github.com/metaist/gdrive-links/blob/main/icons/gdrive-links.png?raw=true",
"homepageTrigger": {
"runFunction": "onHomepage",
"enabled": true
}
},
"drive": {
"onItemsSelectedTrigger": {
"runFunction": "onDriveItemsSelected"
}
}
}
}
Google Cloud Project #
In order to deploy the Workspace Add-on, you need a Google Cloud Project.
- Create a new Google Cloud Console project.
- Configure the OAuth consent screen. Choose
External
if you want to let anyone use this add-on. TheApp name
,User support email
are required.Logo file to upload
is recommended. - If you want to publish this app to the Marketplace, you're going to need to provide a
Privacy Policy
, a YouTube video showing how you use the data, and a written explanation of why you need access to sensitive and/or restricted user data. - Manually add the OAuth scopes:
https://www.googleapis.com/auth/drive.readonly
,https://www.googleapis.com/auth/drive.addons.metadata.readonly
, clickAdd to table
, and thenUpdate
. - Add yourself as a test user and click
Add
. - Go to APIs & Services.
- Click
Enable APIs and Services
, search forDrive
. - Click on
Google Drive API
and thenEnable
. If you don't do this, you'll get a cryptic error message likeException: We're sorry, a server error occurred. Please wait a bit and try again.
when you try to test your add-on.
Test Deploy #
- On the Google Cloud Console upper-right hand corner, click the three dots and then
Project settings
. - Copy the
Project number
. - Go back to the Google Apps Script
Project Settings
and clickChange project
under theGoogle Cloud Platform (GCP) Project
heading. Paste the project number into the box and clickSet project
. If you get any errors, follow the instructions. - Go to the
Editor
and clickRun
in the toolbar. In the popup, clickReview permissions
, choose your account and clickAllow
. Ignore any errors; the goal was just to give the code permission to run. - In the upper-right
Deploy
menu, clickTest deployments
,Install
, and thenDone
. - Open a Google Drive folder and click on an item. In the sidebar, open the Copy Links and select
Authorize Access
. You should now see a link to the item you selected.
Full Deploy #
Now it's time to publish an app. Which then requires you to configure your app in Google Workspace Marketplace SDK. The primary things this involves are having Terms of Service, Privacy Policy, and a video demo of the add-on. I'm also going to need some kind of written description, but I'm hoping this blog post is sufficient.